How to write JS queries for Retool table component
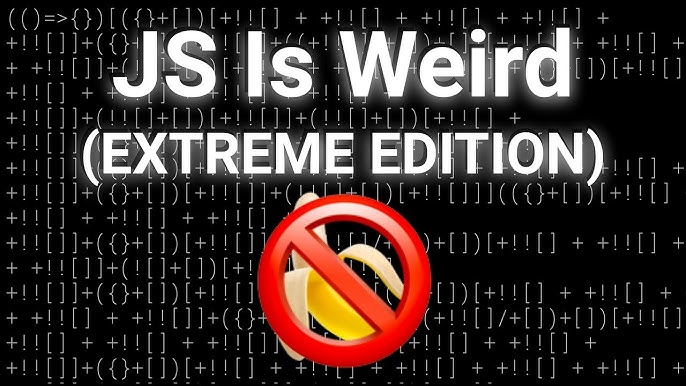
I recently learned from Retool’s community that people struggle to work with Retool’s JS queries. The documentation is not helpful because it shows the method but doesn’t provide a syntax guide. These methods are pretty straightforward, but if you don’t have JS experience, using complex syntax and expressions in the query isn’t easy. Retool’s intellisense provides the syntax help but that info is purely understandable if you have prior experience working with JS.
I tried to provide a guide for almost every available method for the new Retool table’s component.
Before we begin the guide, it is important to know when you need to manipulate the Table’s state programmatically. Normally, the UI provides extensive support for almost every method. But sometimes, you need more granular control over the Table. In that case, this guide can help you write different methods according to your given scenarios.
scrollIntoView(options: any)
As per the Retool’s documentation explanation, this method “Scrolls the canvas or parent container so that the selected component appears in the visible area.”
But what does this mean? Whatever component you’re targeting will become visible on the screen, and the current view will automatically scroll to that component.
This method accepts a option object. In that object you can pass block and behavior property. For block, you’ve four possible values, start, end, nearest, center. And behavior property accepts two types smooth and auto.
Syntax:
table1.scrollIntoView({block:"center",behavior:"smooth"});
table1.scrollIntoView({block:"end",behavior:"auto"});
table1.scrollIntoView({block:"nearest",behavior:"auto"});
table1.scrollIntoView({block:"start",behavior:"auto"});
setHidden(hidden: boolean)
This is one of the simplest methods to hide and show any element in Retool. It accepts a property hidden. You can set it to true or false according to the situation. If you don’t pass the parameter, it will be set to true by default.
Syntax:
table1.setHidden();
table1.setHidden(true);
table1.setHidden(false);
clearChangeset()
This method doesn’t accept any parameters, and you can call it to clear any change-set data or array on a table or any other element.
Syntax:
table1.clearChangeset();
resetFilterStack()
If you have applied some filters to your table and you want to reset those filters via JS query, you can use this method. Remember that this method will only work when you use the table filters, not the custom ones.
Syntax:
table1.resetFilterStack();
clearFilterStack()
This method will clear the Filters applied to the table. But there is a difference between these two tables. If you want to reset the filter, you can use the above method. It will reset the filters to their default state. But if you’re going to clear out all the filters, you can use this method.
Syntax:
table1.clearFilterStack();
clearSelection()
This method clears the selection of Row(s). If you have selected one or more rows in your table and want to clear them out, you can use this method.
Syntax:
table1.clearSelection();
getDisplayedData(options: any)
This method retrieves all of the data in your table. You can also include or exclude the hidden columns. As you can see, I’m using a return statement here. If you don’t use the return statement, then you will not be able to see the output by just calling the method.
Syntax:
return table1.getDisplayedData({includeHiddenColumns:false});
exportData(options: any)
The most used and famous method is exporting Data in Retool. It is used to export the data into a given file type. The available file formats are CSV, TSV, JSON, or Excel. You can also include and exclude the hidden columns in your table.
Syntax:
table1.exportData({fileName:"test",fileType:"csv",includeHiddenColumns:true});
setFilter()
With the SetFilter() method, you can programmatically add more filters to your table. You can also update an existing filter by passing its ID. There is a wide range available for the operator parameter. You can use any of the following values (=,< , >,< = , >= , != , is empty, is not empty) for the operator parameter.
Syntax:
table1.setFilter({columnId:"name",operator:"includes",value:"Lexi"})
// Update exisiting filter with id
table1.setFilter({id:1,columnId:"name",operator:"includes",value:"Lexi"})
setFilterStack(filterStack: any)
This method is powerful when you want to filter your table programmatically in Retool. You can use it to provide different filters and build a stack of filters. You can use the same operator values explained in the SetFilter() method.
Syntax:
// Single Filter
table1.setFilterStack({filters:[{columnId:'name',operator:'includes',value:'Lexi'}]});
// Filter stack
table1.setFilterStack({filters:[{columnId:'name',operator:'includes',value:'Lexi'},{columnId:'name',operator:'includes',value:'Ast'}]});
setSort()
You can sort your table based on a specific column by providing the column information and the sort order. This is helpful when ordering your table based on user input.
Syntax:
table1.setSort({columnId:"id",direction:"desc"});
expandRows() , collapseRows()
This is a new feature in Retool. You can now expand and collapse the Row and add different components under it. But this method will only work when you have the expandRow option enabled.
Syntax:
// Expand row
table1.expandRows({mode:"index",indexType:"display",index:0});
// Collapse row
table1.collapseRows({mode:"index",indexType:"display",index:0});
scrollToRow()
The scroll-to-row method is used to navigate to a particular row inside the table. This method is proper when you have an update operation in Retool and want to highlight that row after the update operation.
Syntax:
// 10 is the row number
table1.scrollToRow({mode:"index",indexType:"display",index:10})
selectRow()
The SelectRow() method selects rows programmatically. This method is suitable for visually showing the user which rows are selected after a particular operation.
Syntax:
// Selects row with key
table1.selectRow({ mode: 'key', key: 4 })
// Selects any visible row
table1.selectRow({mode: 'index', indexType: 'display', index: 4})
// Selects the row that represents table1.data[4]
table1.selectRow({mode: 'index', indexType: 'data', index: 4})
setGrouping(grouping: any)
You can group your rows based on a column and provide a sorting direction based on which you want to group your rows.
Syntax:
table1.setGrouping({columnId:'name',sortDirection:'desc'});
Conculsion
I tried to explain the most used methods of a Retool table. This guide will help you write JS queries in Retool; now, you don’t need to worry about the syntax of these simple methods. Just bookmark this link for any future reference. If you need more help in your Retool development or you’re looking for a freelancer to develop your Internal dashboards using Retool, feel free to contact me.